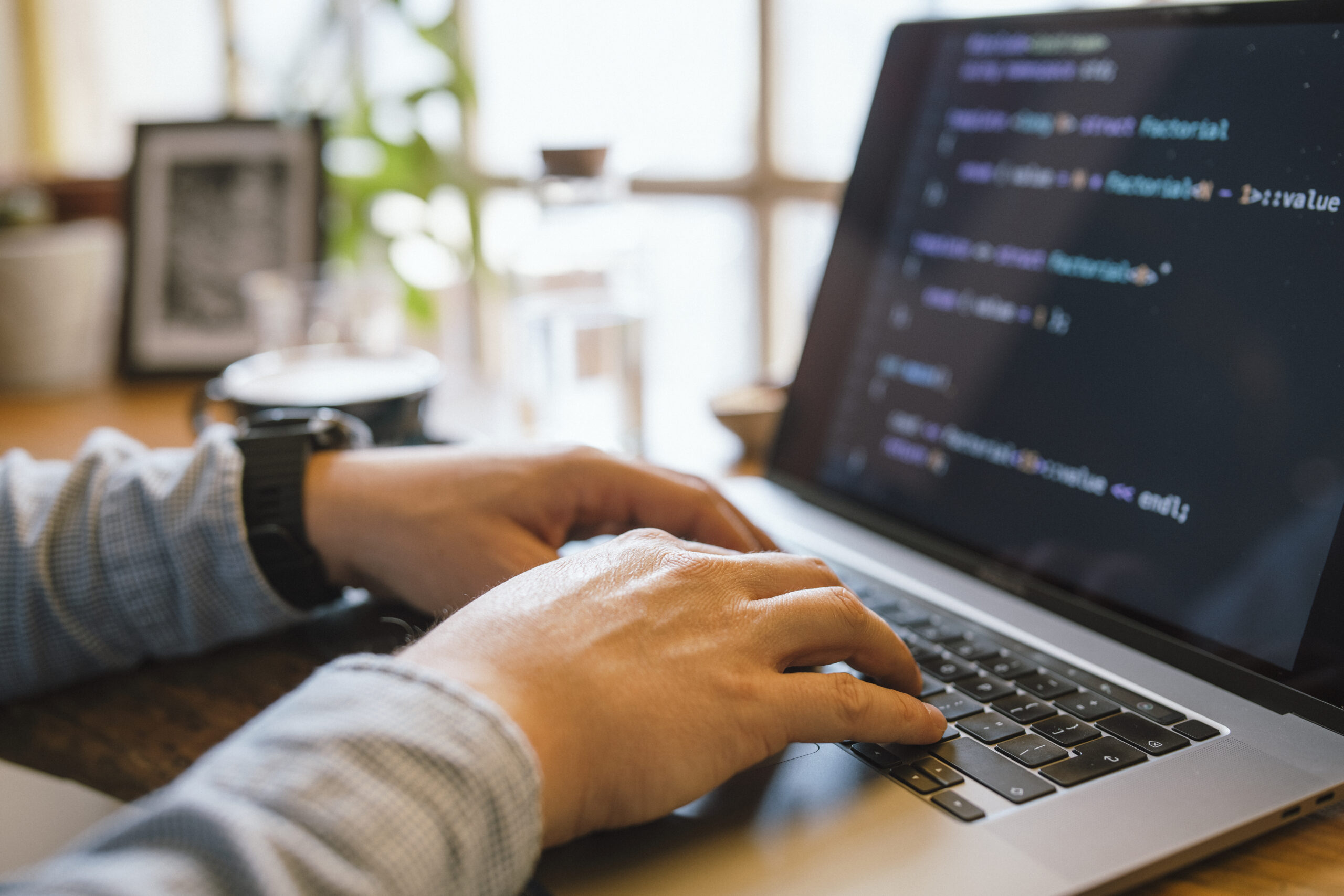
Debugging is Just about the most crucial — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It isn't nearly repairing broken code; it’s about knowledge how and why matters go wrong, and Studying to Feel methodically to resolve difficulties proficiently. No matter if you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can save hours of aggravation and significantly enhance your productivity. Listed here are a number of methods to help builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Resources
One of many quickest methods builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is one part of growth, knowing how you can interact with it effectively throughout execution is Similarly vital. Modern-day growth environments arrive equipped with powerful debugging abilities — but lots of builders only scratch the floor of what these equipment can do.
Consider, by way of example, an Integrated Development Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment help you set breakpoints, inspect the worth of variables at runtime, action by means of code line by line, and in some cases modify code about the fly. When utilised accurately, they Allow you to observe accurately how your code behaves for the duration of execution, that is a must have for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-conclude developers. They enable you to inspect the DOM, keep track of community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can transform aggravating UI challenges into workable tasks.
For backend or process-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command above functioning processes and memory administration. Learning these equipment may have a steeper Finding out curve but pays off when debugging overall performance difficulties, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into comfortable with Edition Regulate units like Git to understand code background, come across the exact second bugs have been released, and isolate problematic changes.
In the end, mastering your instruments usually means going past default options and shortcuts — it’s about producing an personal knowledge of your growth ecosystem to ensure that when problems come up, you’re not missing at the hours of darkness. The greater you are aware of your tools, the greater time you can devote fixing the particular difficulty rather than fumbling through the procedure.
Reproduce the condition
One of the most crucial — and often ignored — actions in efficient debugging is reproducing the trouble. Just before jumping into the code or making guesses, builders will need to produce a regular natural environment or state of affairs wherever the bug reliably seems. With no reproducibility, fixing a bug becomes a game of probability, typically resulting in squandered time and fragile code variations.
The initial step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What steps resulted in The difficulty? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or error messages? The greater depth you have, the a lot easier it turns into to isolate the precise problems below which the bug takes place.
As soon as you’ve collected ample info, seek to recreate the trouble in your neighborhood setting. This could necessarily mean inputting precisely the same data, simulating very similar user interactions, or mimicking procedure states. If the issue seems intermittently, think about producing automated assessments that replicate the sting circumstances or point out transitions involved. These assessments don't just aid expose the condition but additionally protect against regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on selected operating methods, browsers, or underneath particular configurations. Utilizing instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a stage — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You can utilize your debugging equipment far more proficiently, take a look at opportunity fixes properly, and talk a lot more Plainly using your crew or end users. It turns an summary grievance into a concrete challenge — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as direct communications within the process. They typically let you know precisely what happened, where by it took place, and often even why it occurred — if you know how to interpret them.
Get started by looking at the concept cautiously As well as in whole. A lot of developers, specially when underneath time stress, look at the 1st line and right away begin earning assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or perhaps a logic mistake? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and position you towards the accountable code.
It’s also handy to comprehend the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and learning to recognize these can drastically accelerate your debugging course of action.
Some mistakes are obscure or generic, As well as in These scenarios, it’s essential to examine the context where the mistake transpired. Test related log entries, input values, and up to date variations in the codebase.
Don’t neglect compiler or linter warnings both. These normally precede bigger concerns and supply hints about potential bugs.
In the end, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, supporting you pinpoint difficulties faster, decrease debugging time, and become a a lot more productive and self-confident developer.
Use Logging Sensibly
Logging is one of the most powerful resources within a developer’s debugging toolkit. When utilised proficiently, it offers authentic-time insights into how an software behaves, encouraging you comprehend what’s taking place under the hood with no need to pause execution or phase from the code line by line.
A very good logging strategy starts with knowing what to log and at what level. Common logging levels include DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for comprehensive diagnostic details in the course of advancement, Information for common occasions (like successful get started-ups), Alert for likely concerns that don’t break the application, Mistake for genuine difficulties, and FATAL in the event the process can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant knowledge. An excessive amount of logging can obscure important messages and decelerate your program. Concentrate on vital gatherings, state changes, enter/output values, and significant selection points as part of your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace issues in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Enable you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t attainable.
Additionally, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. That has a well-considered-out logging method, you may reduce the time it requires to identify problems, achieve further visibility into your applications, and Enhance the All round maintainability and trustworthiness of your respective code.
Think Just like a Detective
Debugging is not just a specialized endeavor—it's a sort of investigation. To correctly recognize and deal with bugs, builders should technique the method similar to a detective resolving a mystery. This way of thinking aids stop working advanced challenges into manageable components and stick to clues logically to uncover the basis bring about.
Get started by gathering evidence. Look at the signs and symptoms of the challenge: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, acquire just as much suitable information and facts as you can with out jumping to conclusions. Use logs, check instances, and user reviews to piece with each other a clear picture of what’s happening.
Next, variety hypotheses. Ask yourself: What could be causing this actions? Have any variations a short while ago been made towards the codebase? Has this issue happened right before less than very similar conditions? The aim would be to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Attempt to recreate the condition in a very controlled atmosphere. If you suspect a certain perform or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, check with your code queries and let the final results direct you nearer to the reality.
Spend shut focus to small facts. Bugs usually hide while in the least predicted locations—similar to a missing semicolon, an off-by-one mistake, or perhaps a race ailment. Be thorough and affected individual, resisting the urge to patch The problem with no completely comprehending it. Short-term fixes may perhaps hide the actual issue, just for it to resurface afterwards.
And finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for future concerns and assistance Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, technique complications methodically, and turn out to be simpler at uncovering concealed problems in intricate units.
Compose Assessments
Producing exams is among the simplest ways to increase your debugging competencies and overall advancement effectiveness. Assessments not simply help catch bugs early but additionally serve as a safety Internet that provides you self esteem when earning improvements to your codebase. A nicely-tested application is easier to debug since it lets you pinpoint particularly in which and when a difficulty happens.
Begin with unit exams, which give attention to person functions or modules. These small, isolated tests can rapidly reveal whether or not a particular piece of logic is Operating as expected. When a test fails, you immediately know exactly where to appear, considerably reducing some time used debugging. Device exams are Specifically helpful for catching regression bugs—issues that reappear after Beforehand staying fastened.
Up coming, integrate integration checks and conclusion-to-conclude exams into your workflow. These help ensure that many portions of your application work alongside one another efficiently. They’re specifically useful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and less than what problems.
Creating checks also forces you to Assume critically about your code. To check a characteristic adequately, you will need to be familiar with its inputs, predicted outputs, and edge cases. This degree of being familiar with naturally prospects to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. When the exam fails constantly, you could give attention to correcting the bug and watch your examination go when the issue is settled. This strategy makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, producing checks turns debugging from a annoying guessing activity into a structured and predictable method—serving to you capture much more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the problem—looking at your monitor for several hours, attempting Resolution following Option. But Probably the most underrated debugging applications is solely stepping away. Getting breaks can help you reset your intellect, cut down frustration, and often see the issue from a new perspective.
When you're too close to the code for too lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code which you wrote just hours earlier. Within this state, your Mind results in being fewer economical at challenge-fixing. A short walk, a coffee crack, or maybe switching to a unique undertaking for ten–15 minutes can refresh your target. Several developers report finding the root of a dilemma once they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also support avoid burnout, Particularly during for a longer period debugging periods. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away allows you to return with renewed Electrical power and also a clearer attitude. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart tactic. It gives your brain Place to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a psychological puzzle, and relaxation is part of solving it.
Understand From Just about every Bug
Each bug you face is a lot more than just a temporary setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, every one can instruct you something beneficial in case you make the effort to replicate and analyze what went Incorrect.
Commence by inquiring on your own a handful of key concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior tactics like device tests, code assessments, or logging? The answers often reveal blind places in your workflow or understanding and assist you to Develop stronger coding routines shifting forward.
Documenting bugs will also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see patterns—recurring issues or common issues—you can proactively prevent.
In staff environments, sharing Whatever you've realized from a bug with all your friends might be Specially effective. Whether or not it’s through a Slack information, a short write-up, or A fast information-sharing session, assisting Many others stay away from the identical issue boosts staff effectiveness and cultivates a much better Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them check here as critical areas of your development journey. In spite of everything, a number of the best developers are not those who write best code, but those that repeatedly discover from their faults.
In the end, Just about every bug you repair provides a new layer to the talent set. So upcoming time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer on account of it.
Summary
Enhancing your debugging techniques takes time, follow, and tolerance — however the payoff is big. It would make you a more effective, self-confident, and able developer. Another time you're knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.